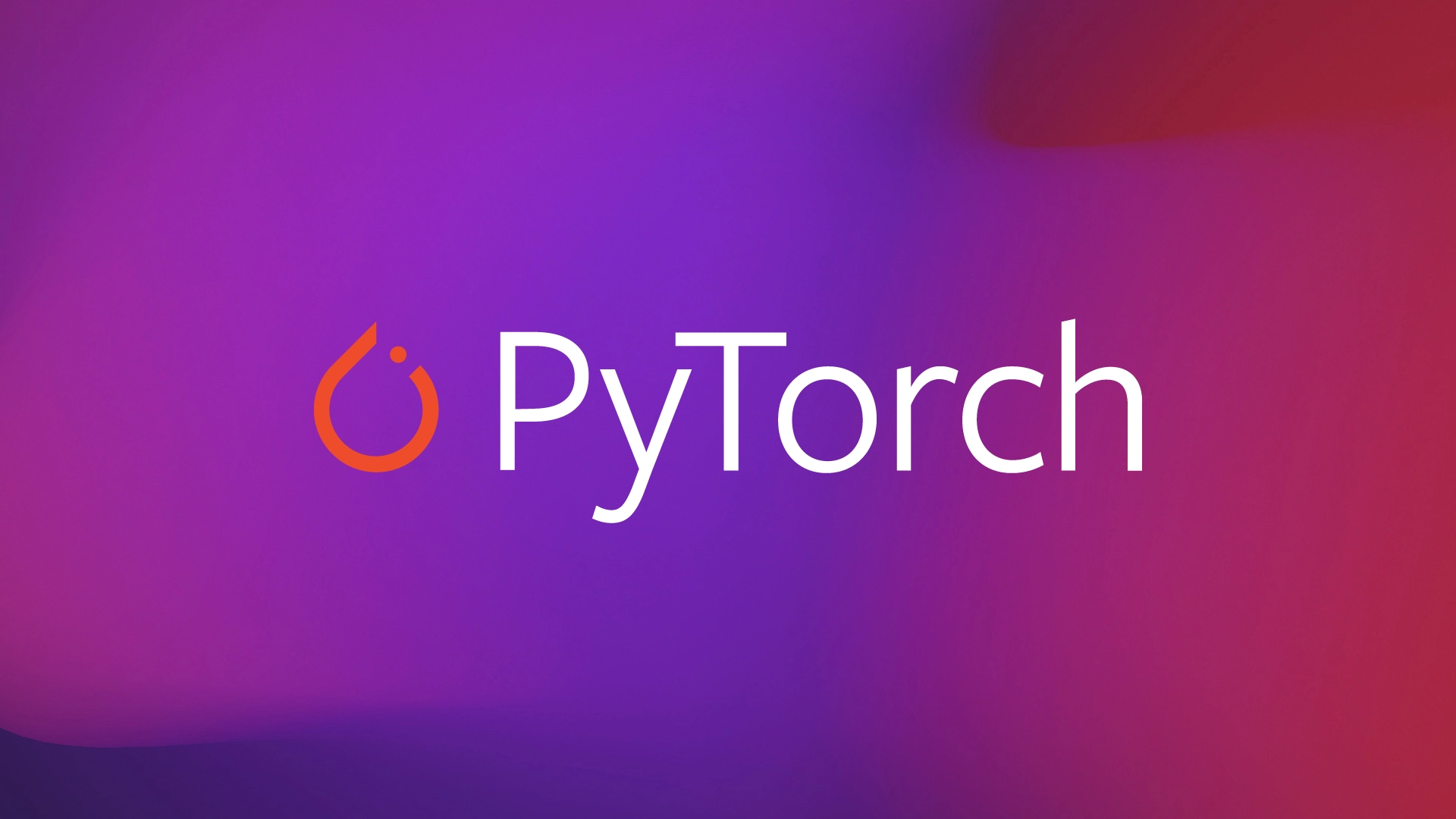
We don't intend to go into the whole "why you should use PyTorch" or "comparing PyTorch vs Tensorflow". Instead we chose to provide a quick reference for actually implementing some real world Deep Learning using PyTorch. Keep in mind each of the featured use cases/tutorials are featured from open source projects, which are constantly under development, and may have different dependencies (versions of Python, CUDA Version, etc.). So please check the documentation thoroughly before implementing. Now enough talk, lets get started shall we?
1. IMAGE RECOGNITION: OBJECT DETECTION USING YOLO V3
Have and idea for an app that uses object detection? Not sure where to start? Luckily, several high quality tutorials exist using PyTorch for implementing the popular YOLO (You Only Look Once) algorithm. YOLO is an object detector that makes use of a fully convolutional neural network to detect an object. YOLOv3 is extremely fast and accurate.
Source: Tumblr, Prosthetic Knowledge
Beginner: A (Very) Minimalist PyTorch implementation of YOLOv3
Perhaps the easiest way to get started is with this minimal implementation, and when we say minimal we mean 9 lines of code. (Credit: github.com/eriklindernoren)
Installation
$ git clone https: //github.com/eriklindernoren/PyTorch-YOLOv3 |
$ cd PyTorch-YOLOv3/ |
$ sudo pip3 install -r requirements.txt |
Download Pre-trained Weights
$ cd weights/ |
$ bash download_weights.sh |
Download COCO
$ cd data/ |
$ bash get_coco_dataset.sh |
Inference
$ python3 detect.py --image_folder /data/samples |
Test
$ python3 test.py --weights_path weights/yolov3.weights |
Source: github.com/eriklindernoren
Advanced: A Deeper Dive Tutorial for Implementing YOLO V3 From Scratch
Let's say you want to get under the hood of YOLO. If you want to understand how to implement this detector by yourself from scratch, you can go through very detailed 5-part tutorial series.This tutorial is perfect for someone who wants to reinforce their PyTorch skills.
The Tutorial Covers the following: (Source: Series: YOLO object detector in PyTorch, Ayoosh Kathuria)
- Part 1 Understanding How YOLO works
- Part 2 : Creating the layers of the network architecture
- Part 3 : Implementing the the forward pass of the network
- Part 4 : Objectness score thresholding and Non-maximum suppression
- Part 5 : Designing the input and the output pipelines
Source: Ayoosh Kathuria, SERIES: YOLO OBJECT DETECTOR IN PYTORCH
We'd be remiss if we didn't mention the original YOLO v3 paper!
Joseph Redmon, Ali Farhadi: YOLOv3: An Incremental Improvement, 2018
2. CHATBOT TUTORIAL
There are several "build your own chatbot" services available out there, while these may be good for quickly deploying a service or function, you're not actually "building" anything. If you want a very deep understanding of to fully harness the power of Natural Language Processing, you may want to actually build it using deep learning. This tutorial from Matthew Inkawhich over at Pytorch.org gives us a great start.
!!!This example requires PyTorch 1.0 or later.
Tutorial Highlights
- Handle loading and pre-processing of Cornell Movie-Dialogs Corpus dataset
- Implement a sequence-to-sequence model with Luong attention mechanism(s)
- Jointly train encoder and decoder models using mini-batches
- Implement greedy-search decoding module
- Interact with trained chatbot
(Sample output pytorch.org)
> hello? bot: hello . > where am I? bot: you re in a hospital . > who are you? bot: i m a lawyer . > how are you doing? bot: i m fine . > are you my friend? bot: no . > you're under arrest bot: i m trying to help you ! > i'm just kidding bot: i m sorry . > where are you from? bot: san francisco . > it's time for me to leave bot: i know . > goodbye bot: goodbye . |
Source: PyTorch.org (https://pytorch.org/tutorials/beginner/chatbot_tutorial.html)
3. DCGAN TUTORIAL: TRAIN A GENERATIVE ADVERSARIAL NETWORK (GAN) TO GENERATE CELEBRITY FACES
We're sure you've seen the "Everybody Dance Now" paper from UC Berkeley, or the DeepFakes that have caused quite a stir, but here is an example (again) from PyTorch.org that allows you to tinker with GAN's, more specifically DCGAN. "A DCGAN is a direct extension of the GAN that it explicitly uses convolutional and convolutional-transpose layers in the discriminator and generator, respectively."
Image Source PyTorch.org "DCGAN Tutorial"
GAN's have several use cases in industry. Learning the fundamentals of this technology will allow you to develop groundbreaking applications. Such applications include but not limited to:
- Font generation
- Anime character generation
- Interactive Image generation
- Text2Image (text to image)
- 3D Object generation
- Image Editing
- Face Aging
- Human Pose Estimation
- Image Inpainting (hole filling)
- High-resolution image generation
- Adversarial Examples
- Visual Saliency Prediction
- Object Detection/Recognition
- Robotics
- Video (generation/prediction)
- Synthetic Data Generation
Find more here
Source PyTorch.org "DCGAN Tutorial" https://pytorch.org/tutorials/beginner/dcgan_faces_tutorial.html
That's it, we hope to have given you a few projects to kickstart your AI development in PyTorch. Let us know how it goes in the comments below or on social media!
Facebook: https://www.facebook.com/exxactcorp/
Twitter: https://twitter.com/Exxactcorp
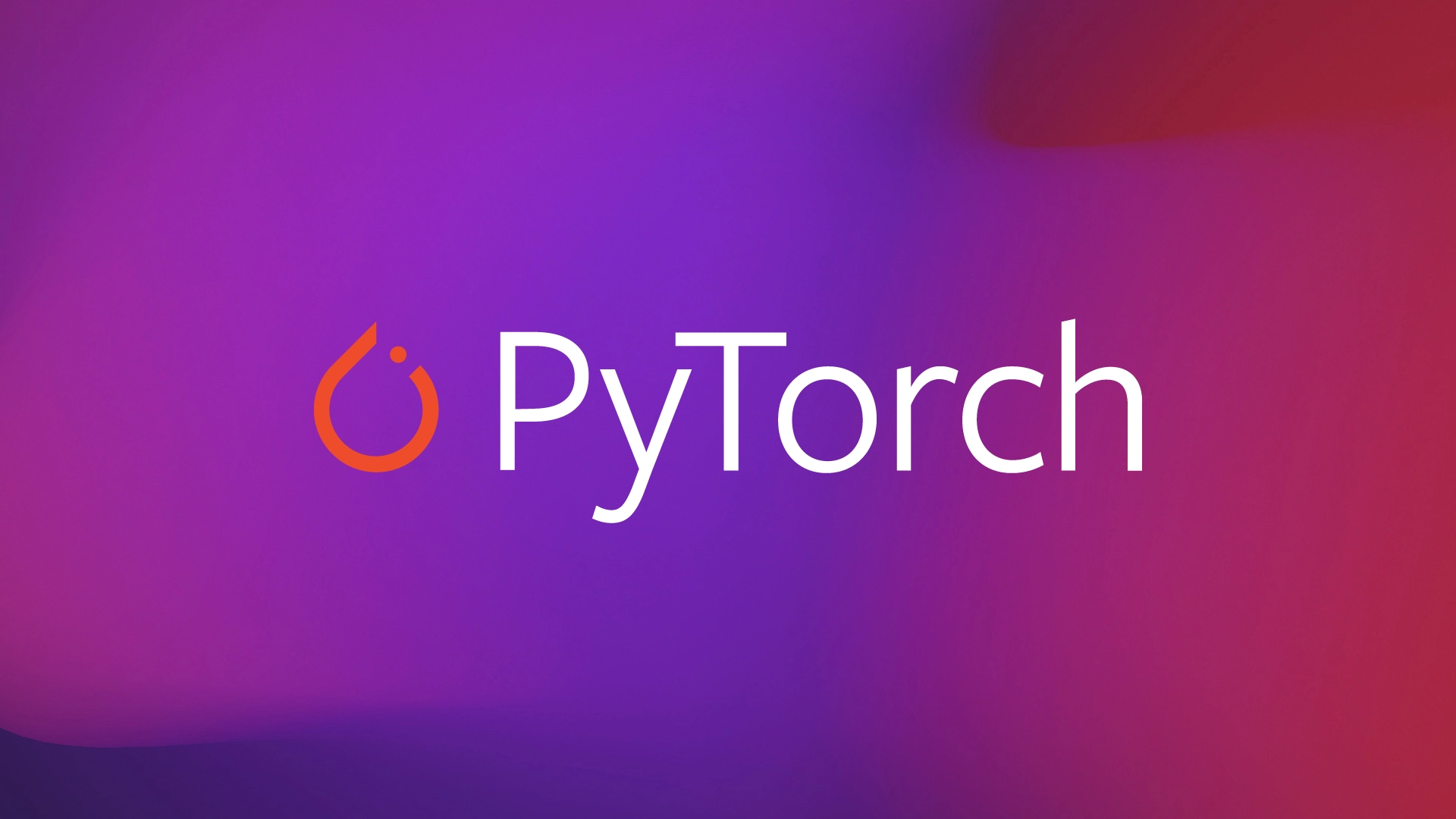
Kickstart Your Deep Learning With These 3 PyTorch Projects
We don't intend to go into the whole "why you should use PyTorch" or "comparing PyTorch vs Tensorflow". Instead we chose to provide a quick reference for actually implementing some real world Deep Learning using PyTorch. Keep in mind each of the featured use cases/tutorials are featured from open source projects, which are constantly under development, and may have different dependencies (versions of Python, CUDA Version, etc.). So please check the documentation thoroughly before implementing. Now enough talk, lets get started shall we?
1. IMAGE RECOGNITION: OBJECT DETECTION USING YOLO V3
Have and idea for an app that uses object detection? Not sure where to start? Luckily, several high quality tutorials exist using PyTorch for implementing the popular YOLO (You Only Look Once) algorithm. YOLO is an object detector that makes use of a fully convolutional neural network to detect an object. YOLOv3 is extremely fast and accurate.
Source: Tumblr, Prosthetic Knowledge
Beginner: A (Very) Minimalist PyTorch implementation of YOLOv3
Perhaps the easiest way to get started is with this minimal implementation, and when we say minimal we mean 9 lines of code. (Credit: github.com/eriklindernoren)
Installation
$ git clone https: //github.com/eriklindernoren/PyTorch-YOLOv3 |
$ cd PyTorch-YOLOv3/ |
$ sudo pip3 install -r requirements.txt |
Download Pre-trained Weights
$ cd weights/ |
$ bash download_weights.sh |
Download COCO
$ cd data/ |
$ bash get_coco_dataset.sh |
Inference
$ python3 detect.py --image_folder /data/samples |
Test
$ python3 test.py --weights_path weights/yolov3.weights |
Source: github.com/eriklindernoren
Advanced: A Deeper Dive Tutorial for Implementing YOLO V3 From Scratch
Let's say you want to get under the hood of YOLO. If you want to understand how to implement this detector by yourself from scratch, you can go through very detailed 5-part tutorial series.This tutorial is perfect for someone who wants to reinforce their PyTorch skills.
The Tutorial Covers the following: (Source: Series: YOLO object detector in PyTorch, Ayoosh Kathuria)
- Part 1 Understanding How YOLO works
- Part 2 : Creating the layers of the network architecture
- Part 3 : Implementing the the forward pass of the network
- Part 4 : Objectness score thresholding and Non-maximum suppression
- Part 5 : Designing the input and the output pipelines
Source: Ayoosh Kathuria, SERIES: YOLO OBJECT DETECTOR IN PYTORCH
We'd be remiss if we didn't mention the original YOLO v3 paper!
Joseph Redmon, Ali Farhadi: YOLOv3: An Incremental Improvement, 2018
2. CHATBOT TUTORIAL
There are several "build your own chatbot" services available out there, while these may be good for quickly deploying a service or function, you're not actually "building" anything. If you want a very deep understanding of to fully harness the power of Natural Language Processing, you may want to actually build it using deep learning. This tutorial from Matthew Inkawhich over at Pytorch.org gives us a great start.
!!!This example requires PyTorch 1.0 or later.
Tutorial Highlights
- Handle loading and pre-processing of Cornell Movie-Dialogs Corpus dataset
- Implement a sequence-to-sequence model with Luong attention mechanism(s)
- Jointly train encoder and decoder models using mini-batches
- Implement greedy-search decoding module
- Interact with trained chatbot
(Sample output pytorch.org)
> hello? bot: hello . > where am I? bot: you re in a hospital . > who are you? bot: i m a lawyer . > how are you doing? bot: i m fine . > are you my friend? bot: no . > you're under arrest bot: i m trying to help you ! > i'm just kidding bot: i m sorry . > where are you from? bot: san francisco . > it's time for me to leave bot: i know . > goodbye bot: goodbye . |
Source: PyTorch.org (https://pytorch.org/tutorials/beginner/chatbot_tutorial.html)
3. DCGAN TUTORIAL: TRAIN A GENERATIVE ADVERSARIAL NETWORK (GAN) TO GENERATE CELEBRITY FACES
We're sure you've seen the "Everybody Dance Now" paper from UC Berkeley, or the DeepFakes that have caused quite a stir, but here is an example (again) from PyTorch.org that allows you to tinker with GAN's, more specifically DCGAN. "A DCGAN is a direct extension of the GAN that it explicitly uses convolutional and convolutional-transpose layers in the discriminator and generator, respectively."
Image Source PyTorch.org "DCGAN Tutorial"
GAN's have several use cases in industry. Learning the fundamentals of this technology will allow you to develop groundbreaking applications. Such applications include but not limited to:
- Font generation
- Anime character generation
- Interactive Image generation
- Text2Image (text to image)
- 3D Object generation
- Image Editing
- Face Aging
- Human Pose Estimation
- Image Inpainting (hole filling)
- High-resolution image generation
- Adversarial Examples
- Visual Saliency Prediction
- Object Detection/Recognition
- Robotics
- Video (generation/prediction)
- Synthetic Data Generation
Find more here
Source PyTorch.org "DCGAN Tutorial" https://pytorch.org/tutorials/beginner/dcgan_faces_tutorial.html
That's it, we hope to have given you a few projects to kickstart your AI development in PyTorch. Let us know how it goes in the comments below or on social media!
Facebook: https://www.facebook.com/exxactcorp/
Twitter: https://twitter.com/Exxactcorp